What Is Controller Advice In Spring Boot?
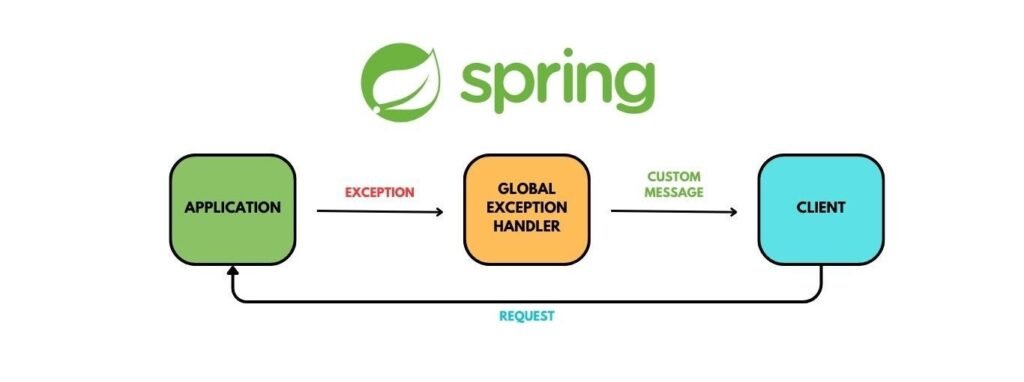
@ControllerAdvice
is an annotation in Spring Boot global exception handler used to define and model attributes that apply to all or selected controllers in your application. It allows you to centralize exception handling logic and reuse it across multiple controllers.
When you annotate a class with @ControllerAdvice
, it becomes a global exception handler, meaning it can intercept exceptions thrown by any controller within your application. You can define methods within this class annotated with @ExceptionHandler
to handle specific types of exceptions. These methods can return appropriate responses or perform actions like logging errors.
Additionally, you can use @ControllerAdvice
to define model attributes that should be added to all model objects returned by your controllers. This can be useful for adding common attributes like user authentication details or application configuration settings.
Overall, @ControllerAdvice
provides a powerful mechanism for centralizing exception handling and model attribute management in Spring Boot applications.
Exception Handling In Spring Boot(Spring Boot Global Exception Handler)
Exception handling in Spring Boot allows you to manage errors gracefully, providing a way to handle unexpected situations that may occur during the execution of your application. Here’s a brief explanation along with a coding example:
Define Custom Exception Classes: Create custom exception classes to represent different types of errors that can occur in your application. These classes can extend from RuntimeException
or any other appropriate exception class.
public class ResourceNotFoundException extends RuntimeException {
public ResourceNotFoundException(String message) {
super(message);
}
}
public class BadRequestException extends RuntimeException {
public BadRequestException(String message) {
super(message);
}
}
Global Exception Handling: In Spring Boot, you can define a global exception handler to catch and handle exceptions thrown by your controllers or services
@ControllerAdvice
public class LearnSpringBootOnlineExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public ResponseEntity<Object> handleResourceNotFoundException(ResourceNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
@ExceptionHandler(BadRequestException.class)
@ResponseStatus(HttpStatus.BAD_REQUEST)
public ResponseEntity<Object> handleBadRequestException(BadRequestException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.BAD_REQUEST);
}
// Handle other exceptions if needed
}
Throwing Exceptions: Throw custom exceptions from your controller or service layer when necessary.
@RestController
public class LearnSpringBootOnlineController {
@Autowired
private LearnSpringBootOnlineService learnSpringBootOnlineService;
@GetMapping("/api/resource/{id}")
public ResponseEntity<Resource> getResource(@PathVariable Long id) {
Resource resource = learnSpringBootOnlineService.findResourceById(id);
if (resource == null) {
throw new ResourceNotFoundException("Resource not found with id: " + id);
}
return ResponseEntity.ok(resource);
}
@PostMapping("/api/resource")
public ResponseEntity<Void> createResource(@RequestBody Resource resource) {
if (resource == null || resource.getName() == null) {
throw new BadRequestException("Invalid resource provided");
}
learnSpringBootOnlineService.saveResource(resource);
return ResponseEntity.status(HttpStatus.CREATED).build();
}
// Other controller methods
}
In this example, ResourceNotFoundException
and BadRequestException
are custom exceptions that extend RuntimeException
. We have a GlobalExceptionHandler
class annotated with @ControllerAdvice
which contains methods annotated with @ExceptionHandler
to handle specific types of exceptions. These methods return appropriate HTTP status codes along with error messages.
In the controller, we throw these custom exceptions when certain conditions are not met, such as when a requested resource is not found or when invalid data is provided for resource creation.
This setup ensures that exceptions are handled uniformly across your application, providing meaningful responses to clients when errors occur.
Explore our diverse collection of blogs covering a wide range of topics here