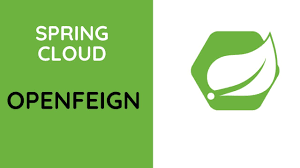
image credits : https://spring.io/
Table of Contents
Overview
Feign Client with Spring Boot allows you to easily consume RESTful web services by defining interfaces with annotated methods.
Feign Client is a useful tool in the Spring ecosystem for several reasons:
- Declarative REST Client: Feign Client allows developers to define RESTful API integrations using a declarative interface rather than low-level HTTP client implementations. This makes the code more readable, concise, and easier to maintain.
- Reduced Boilerplate Code: By using Feign Client, developers can avoid writing repetitive code for creating HTTP requests, handling responses, and error handling. Feign handles these tasks behind the scenes, reducing boilerplate code and making development more efficient.
- Integration with Spring Ecosystem: Feign seamlessly integrates with other components of the Spring ecosystem, such as Spring Boot and Spring Cloud. This integration simplifies configuration and allows for easy integration with service discovery, load balancing, and circuit breaking mechanisms provided by Spring Cloud.
- Load Balancing and Fault Tolerance: Feign Client integrates with Ribbon, a client-side load balancer, allowing for automatic load balancing across multiple instances of a service. Additionally, Feign supports integration with Hystrix for implementing fault tolerance and fallback mechanisms, improving the resilience of microservices architectures.
- Annotation-Based Configuration: Feign Client leverages annotations such as
@FeignClient
,@RequestMapping
, and@RequestParam
to define API contracts and parameters. This annotation-based approach simplifies the configuration and reduces the learning curve for developers familiar with Spring. - Dynamic Proxy Generation: Feign Client dynamically generates proxy classes at runtime based on the annotated interfaces. This dynamic proxy generation enables runtime method invocation for making HTTP requests, allowing developers to focus on defining API contracts rather than implementing HTTP client logic.
- Interoperability: Feign Client supports various serialization formats such as JSON, XML, and form-encoded data, making it compatible with a wide range of RESTful APIs. It also supports integration with custom error decoders and request interceptors, providing flexibility for handling specific API requirements.
Below is a step-by-step guide on how to use Feign Client in a Spring Boot application
Add Feign Client Dependency
Make sure you have the necessary dependencies in your pom.xml
or build.gradle
file:
For Maven:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
For Gradle:
implementation 'org.springframework.cloud:spring-cloud-starter-openfeign'
Enable Feign Client in Spring Boot Application
Annotate your main Spring Boot application class with @EnableFeignClients
to enable Feign Client functionality:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.openfeign.EnableFeignClients;
@SpringBootApplication
@EnableFeignClients
public class LearnSpringBootOnlineApplication {
public static void main(String[] args) {
SpringApplication.run(LearnSpringBootOnlineApplication.class, args);
}
}
Define Feign Client Interface
Create an interface that declares the remote RESTful service’s endpoints using Spring annotations such as @FeignClient
, @RequestMapping
, etc. For example:
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
@FeignClient(name = "learn-spring-boot-online-service", url = "https://learnspringbootonline.com")
public interface LearnSpringBootOnlineFeignClient {
@GetMapping("/api/resource")
String getResource();
}
Inject Feign Client in Your Service or Controller
Inject the Feign Client interface into your service or controller where you want to consume the RESTful service:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class LearnSpringBootOnlineService {
private final LearnSpringBootOnlineFeignClient feignClient;
@Autowired
public LearnSpringBootOnlineService(LearnSpringBootOnlineFeignClient feignClient) {
this.feignClient = feignClient;
}
public String getRemoteResource() {
return feignClient.getResource();
}
}
Enable Feign Client Logging
To enable logging for Feign Client, you can configure it using Spring Boot’s application properties or YAML file:
logging.level.<feign-client-package>=<log-level>
For example, to set the logging level for all Feign clients to DEBUG, add the following line to your application.properties
or application.yml
file:
logging.level.feign=DEBUG
Additionally, you can specify logging levels for specific Feign clients by replacing <feign-client-package>
with the package name of your Feign client interface.
Run Your Spring Boot Application
Finally, run your Spring Boot application, and Feign Client will handle the communication with the remote RESTful service. Log entries for Feign Client operations will be displayed according to the logging configuration you’ve set
That’s it! You’ve successfully configured and used Feign Client in your Spring Boot application, along with enabling logging for Feign Client operations.
Conclusion
In summary, Feign Client is needed because it simplifies the development of RESTful API integrations by providing a declarative and annotation-based approach, reducing boilerplate code, and seamlessly integrating with the Spring ecosystem for enhanced scalability, resilience, and maintainability of microservices architectures.
Explore our diverse collection of blogs covering a wide range of topics here