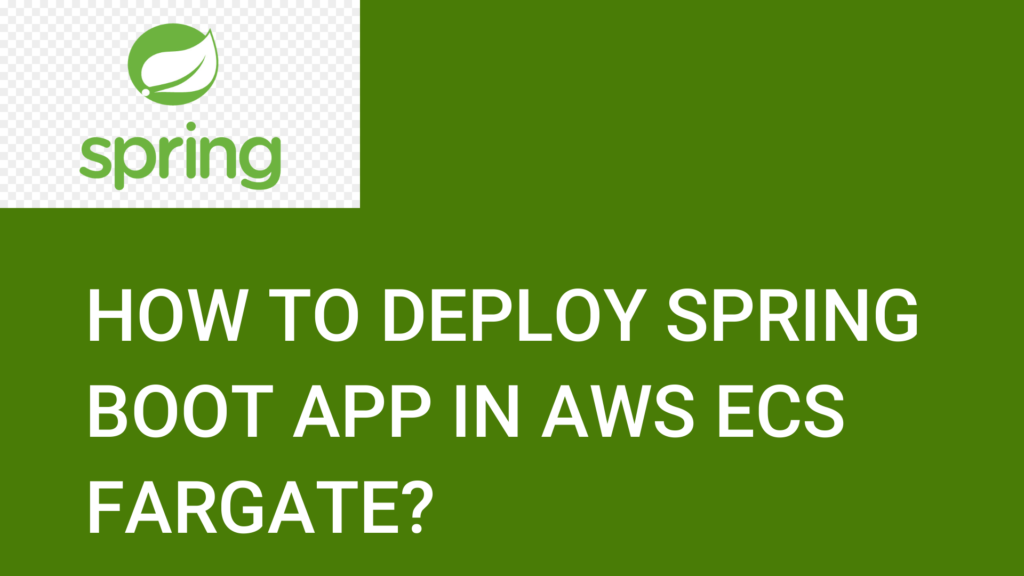
Table of Contents
Overview
AWS ECS (Elastic Container Service) Fargate is a serverless container management service provided by Amazon Web Services (AWS). It allows you to run Docker containers without having to manage the underlying infrastructure. Here’s what AWS ECS Fargate offers:
- Serverless Container Management: With Fargate, you don’t need to provision or manage servers. AWS takes care of the underlying infrastructure, allowing you to focus on deploying and managing your containers.
- Container Orchestration: ECS Fargate enables you to orchestrate the scheduling and execution of your containers. You can define task definitions that specify how your containers should run, including CPU and memory requirements, networking configuration, and container images.
- Scalability: Fargate automatically scales your containers based on workload demands. You can configure autoscaling policies to adjust the number of containers running your application in response to changes in traffic or resource utilization.
- Isolation and Security: Each container in Fargate runs in its own isolated environment, providing enhanced security and ensuring that issues in one container don’t impact others. Fargate also integrates with AWS IAM for fine-grained access control.
- Integration with AWS Services: ECS Fargate seamlessly integrates with other AWS services such as Elastic Load Balancing, AWS Auto Scaling, AWS CloudWatch, and AWS Identity and Access Management (IAM). This allows you to leverage the full capabilities of the AWS ecosystem for managing, monitoring, and securing your containers.
- Pay-as-you-go Pricing: Fargate operates on a pay-as-you-go pricing model. You only pay for the compute resources your containers consume, which can be more cost-effective compared to traditional virtual machine-based deployments where you have to pay for the entire VM regardless of the utilization.
Overall, AWS ECS Fargate provides a serverless, scalable, and secure platform for running containerized workloads in the AWS cloud, enabling you to focus on building and deploying applications without worrying about infrastructure management.
Steps To Deploy Spring Boot App In AWS ECS Fargate
Deploying a Spring Boot application into AWS ECS Fargate with autoscaling involves several steps. Here’s a general guide on how to achieve this:
- Containerize your Spring Boot application: First, you need to containerize your Spring Boot application using Docker. This involves creating a Dockerfile that describes how to build your application into a Docker image.
- Push Docker image to a container registry: Once you have created the Docker image, you need to push it to a container registry such as Amazon ECR (Elastic Container Registry) or Docker Hub. This allows ECS to pull the image when deploying your application.
- Create an ECS cluster: In the AWS Management Console, navigate to ECS and create a new cluster. Choose the Fargate launch type for your cluster.
- Create a task definition: Define a task definition that specifies how your container should run in ECS. This includes specifying the Docker image to use, CPU and memory requirements, networking configuration, etc.
- Set up an ECS service: Create an ECS service within your cluster and specify the task definition you created earlier. Configure the service to use Fargate launch type and set up desired task count and autoscaling parameters.
- Configure autoscaling: In the ECS service configuration, enable autoscaling and define scaling policies based on metrics such as CPU utilization or memory utilization. This allows ECS to automatically scale the number of tasks running based on the specified conditions.
- Set up load balancing: If your application needs to be accessible from the internet, configure a load balancer such as Application Load Balancer (ALB) or Network Load Balancer (NLB) to distribute traffic to your ECS tasks.
- Configure DNS: Optionally, configure a DNS record to point to the load balancer’s endpoint to make your application accessible via a custom domain name.
- Testing and monitoring: Test your deployment to ensure everything is working as expected. Monitor your ECS service and autoscaling activity to ensure that it’s scaling appropriately based on the configured metrics.
Implementation
Containerize your Spring Boot application:
Assuming you have a Spring Boot application with a Dockerfile
like this:
FROM openjdk:11-jre-slim
COPY target/my-spring-app.jar /app/my-spring-app.jar
CMD ["java", "-jar", "/app/my-spring-app.jar"]
Push Docker image to a container registry:
docker build -t my-spring-app .
docker tag my-spring-app:latest <your-registry-url>/my-spring-app:latest
docker push <your-registry-url>/my-spring-app:latest
Create an ECS cluster ,Task Definition, ECS Service, Load Balancer and Auto Scaling:
- Install Terraform: If you haven’t already installed Terraform, you can download it from the official website: Terraform Downloads. Follow the installation instructions for your operating system.
- Set up AWS credentials: Configure your AWS credentials either by setting environment variables or using the AWS CLI
aws configure
command. - Write Terraform Configuration:a. Create a file named
main.tf
and define the AWS provider and ECS resources
provider "aws" {
region = "us-east-1" # Update with your desired region
}
resource "aws_ecs_cluster" "my_cluster" {
name = "my-ecs-cluster"
}
resource "aws_ecs_task_definition" "my_task_definition" {
family = "my-spring-boot-task"
container_definitions = <<TASK_DEFINITION
[
{
"name": "my-spring-boot-container",
"image": "<your-registry-url>/my-spring-boot-app:latest",
"cpu": 256,
"memory": 512,
"essential": true,
"portMappings": [
{
"containerPort": 8080,
"protocol": "tcp"
}
]
}
]
TASK_DEFINITION
}
resource "aws_ecs_service" "my_service" {
name = "my-spring-boot-service"
cluster = aws_ecs_cluster.my_cluster.id
task_definition = aws_ecs_task_definition.my_task_definition.arn
desired_count = 2
launch_type = "FARGATE"
network_configuration {
subnets = ["subnet-12345678"] # Update with your subnet IDs
security_groups = ["sg-12345678"] # Update with your security group IDs
assign_public_ip = true
}
capacity_provider_strategy {
capacity_provider = "FARGATE"
weight = 1
}
load_balancer {
target_group_arn = "arn:aws:elasticloadbalancing:us-east-1:123456789012:targetgroup/my-target-group/1234567890abcdef"
container_name = "my-spring-boot-container"
container_port = 8080
}
lifecycle {
ignore_changes = [desired_count]
}
deployment_controller {
type = "ECS"
}
# Autoscaling configuration
depends_on = [aws_appautoscaling_target.my_target]
}
resource "aws_appautoscaling_target" "my_target" {
max_capacity = 10
min_capacity = 2
resource_id = "service/my-ecs-cluster/my-spring-boot-service"
scalable_dimension = "ecs:service:DesiredCount"
service_namespace = "ecs"
}
resource "aws_appautoscaling_policy" "my_policy" {
name = "my-scaling-policy"
policy_type = "TargetTrackingScaling"
resource_id = aws_appautoscaling_target.my_target.resource_id
scalable_dimension = aws_appautoscaling_target.my_target.scalable_dimension
service_namespace = aws_appautoscaling_target.my_target.service_namespace
target_tracking_scaling_policy_configuration {
predefined_metric_specification {
predefined_metric_type = "ECSServiceAverageCPUUtilization"
}
target_value = 50.0
}
}
- Initialize Terraform: Run
terraform init
in the directory containing your Terraform configuration files. This command initializes Terraform and downloads necessary provider plugins. - Review and Apply Changes: Run
terraform plan
to review the changes that Terraform will apply, and thenterraform apply
to apply those changes. - Verify: After Terraform applies the changes, verify the resources in the AWS Management Console or using AWS CLI commands.
Testing and monitoring:
Test your deployment to ensure it’s working as expected. Monitor your ECS service and autoscaling activity in the AWS Management Console or using CloudWatch.
Conclusion
In conclusion, deploying a Spring Boot application into AWS ECS Fargate with autoscaling using Terraform involves several steps:
- Containerize your Spring Boot application by creating a Dockerfile and building a Docker image.
- Write Terraform configuration to define ECS resources such as clusters, task definitions, services, and autoscaling policies.
- Initialize Terraform in your project directory and review the planned changes using
terraform plan
. - Apply the Terraform configuration using
terraform apply
to create the ECS infrastructure and enable autoscaling for your Spring Boot application.
By following these steps, you can efficiently deploy and manage your Spring Boot application on AWS ECS Fargate, ensuring scalability and reliability while automating the infrastructure setup using Terraform. Adjustments can be made to the configuration to meet specific requirements and preferences.
Explore our diverse collection of blogs covering a wide range of topics here