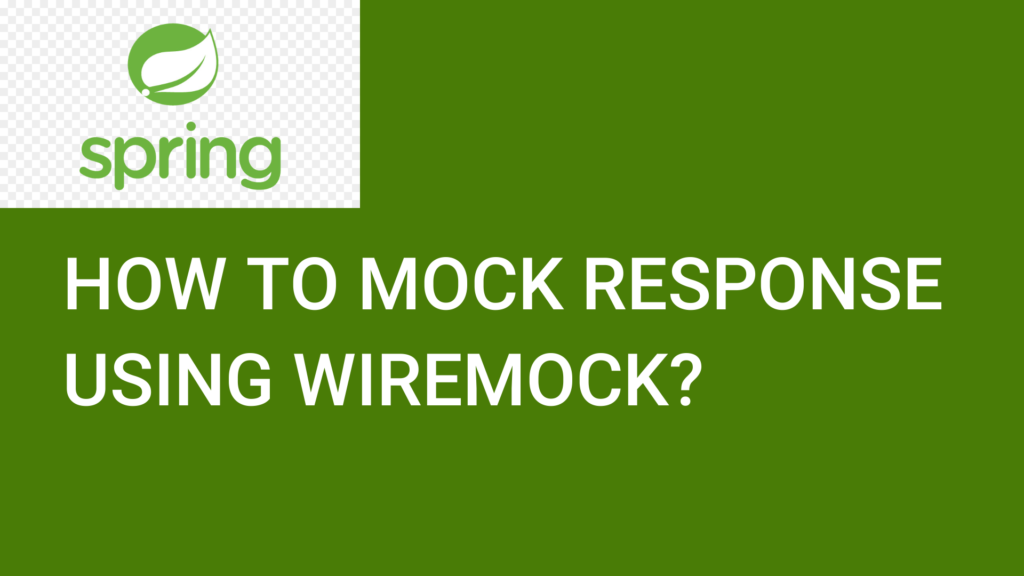
Table of Contents
Introduction For WireMock
WireMock is a flexible library for stubbing and mocking HTTP services. It allows you to simulate HTTP responses from external services during testing or development, enabling you to isolate your application from dependencies on external systems. In the context of Spring Boot, you can easily integrate WireMock to mock external service responses.
API mocking involves creating a simple simulation of an API, accepting the same types of request and returning identically structured responses as the real thing, enabling fast and reliable development and testing. API mocking is typically used during development and testing as it allows you to build your app without worrying about 3rd party APIs or sandboxes breaking. It can also be used to rapidly prototype APIs that don’t exist yet.
Add Dependency
For Maven:
<dependency>
<groupId>com.github.tomakehurst</groupId>
<artifactId>wiremock</artifactId>
<version>2.27.2</version> <!-- Replace with the latest version -->
<scope>test</scope> <!-- Ensure WireMock is only included in test scope -->
</dependency>
For Gradle:
dependencies {
testImplementation 'com.github.tomakehurst:wiremock:2.27.2'
}
Implementation
In your test resources directory (src/test/resources
/wiremock), create a directory named mappings
. Inside this directory, you can define JSON files to configure the responses you want WireMock to return. For example, you can create a file named example_mapping.json
with the following content:
{
"request": {
"method": "GET",
"url": "/learnspringbootonline"
},
"response": {
"status": 200,
"bodyFileName": "responses/example_response.json
}
}
This JSON configuration specifies that when WireMock receives a GET request to /learnspringbootonline
, it should respond with a 200 status code and the body file example_response.json.
In your test resources directory (src/test/resources
/wiremock), create a response directory named __files/responses. Inside this directory, you can define JSON files to configure the response body you want WireMock to return. For example, you can create a file named example_response.json
with the following content:
{
"name": "Learn Spring Boot Online",
"url": "https://learnspringbootonline.com/"
}
In your JUnit or TestNG test classes, you need to initialize WireMock before running your tests. You can use annotations like @BeforeClass
or @Before
to set up WireMock. Here’s an example using JUnit 5:
import com.github.tomakehurst.wiremock.WireMockServer;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.BeforeAll;
public class LearnSpringBootOnlineTest {
private static WireMockServer wireMockServer;
@BeforeAll
public static void setup() {
wireMockServer = new WireMockServer();
wireMockServer.start();
}
@AfterAll
public static void teardown() {
wireMockServer.stop();
}
// Your test methods go here
}
In your test methods, configure your Spring Boot application to use WireMock’s URL instead of the real external service URL. You can typically do this by setting properties or environment variables. For example:
@SpringBootTest(properties = {"external.service.url=http://localhost:8080"})
public class LearnSpringBootOnlineTest {
@Autowired
private LearnSpringBootOnlineService learnSpringBootOnlineService;
// Your test methods go here
}
Now, when you run your tests, WireMock will intercept HTTP requests to the configured URLs and respond with the configured mock responses.
That’s it! You’ve now set up WireMock for mocking responses in your Spring Boot application. This approach allows you to effectively test your application’s behavior without relying on external services.
Conclusion
In conclusion, WireMock is a valuable tool for mocking HTTP services in Spring Boot applications, facilitating efficient testing and development workflows. By adding WireMock as a test dependency, configuring mock responses through mappings, initializing the WireMock server in tests, and directing application requests to the WireMock server, developers can create controlled testing environments independent of external services. This approach ensures reliable and predictable testing outcomes, ultimately leading to improved software quality and faster development cycles.
Explore our diverse collection of blogs covering a wide range of topics here.