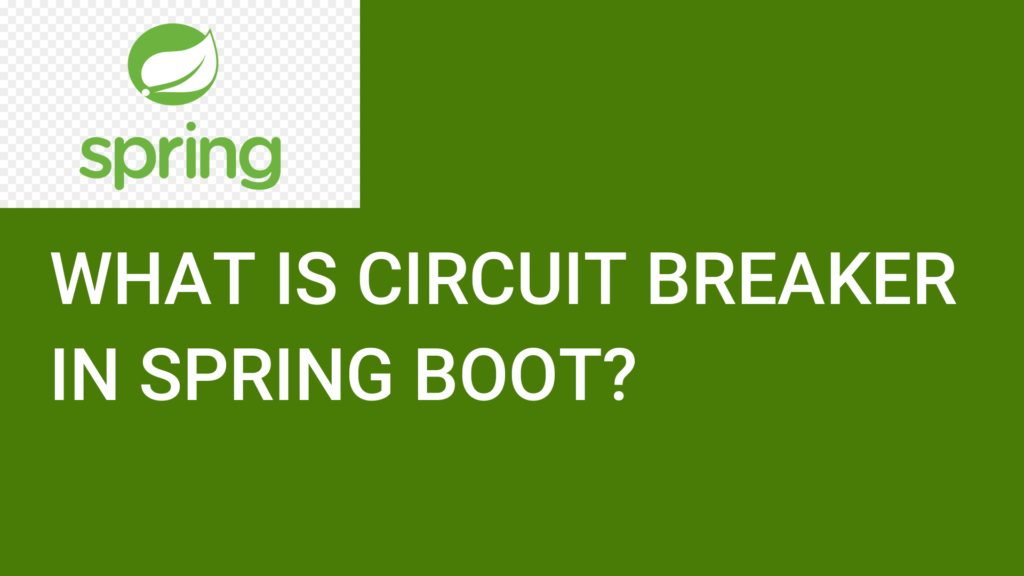
Table of Contents
Overview Of Circuit Breaker In Spring Boot
In Spring Boot, a circuit breaker is a design pattern used to handle and prevent failures in distributed systems by providing a mechanism to detect and handle failures when calling remote services. It aims to improve the stability and resilience of the application by isolating and controlling the impact of failures.
The basic idea behind a circuit breaker is similar to an electrical circuit breaker. When there is a failure in a remote service (such as a timeout or an exception), the circuit breaker “opens” and prevents further calls to the failing service for a predefined period. During this period, the circuit breaker can redirect calls to a fallback method or return predefined default values instead of failing completely. After the predefined period, the circuit breaker “closes” again and allows calls to the remote service, effectively “resetting” the circuit.
States In Circuit Breaker
In a circuit breaker pattern, there are typically three main states that the circuit breaker can be in:
- Closed: In the closed state, the circuit breaker allows requests to pass through to the remote service as usual. This means that all requests are executed normally without any intervention from the circuit breaker. The circuit breaker monitors the responses from the remote service during this state. If the failure threshold (e.g., number of consecutive failures or failure rate) is not exceeded, the circuit breaker remains in the closed state.
- Open: When the failure threshold is exceeded, indicating that the remote service may be experiencing issues, the circuit breaker transitions to the open state. In this state, the circuit breaker prevents requests from being forwarded to the remote service. Instead, it immediately redirects requests to a fallback mechanism or returns predefined default responses. This prevents further calls to the failing service and gives it time to recover.
- Half-Open: After a predefined time period (often referred to as the “reset timeout”), the circuit breaker transitions from the open state to the half-open state. In this state, the circuit breaker allows a limited number of requests to pass through to the remote service. The purpose of the half-open state is to test whether the remote service has recovered and is able to handle requests successfully again. If these test requests succeed, the circuit breaker transitions back to the closed state, allowing normal operation to resume. However, if any of these test requests fail, the circuit breaker returns to the open state, indicating that the remote service is still experiencing issues.
These three states—closed, open, and half-open—enable the circuit breaker to dynamically adapt to the health and availability of the remote service, providing resilience and fault tolerance in distributed systems.
Add Dependency
Spring Boot provides integration with circuit breakers through libraries like Netflix’s Hystrix or Resilience4j. Here’s an example using Resilience4j:
First, you need to add the Resilience4j dependency to your pom.xml
or build.gradle
file:
For Maven:
<dependency>
<groupId>io.github.resilience4j</groupId>
<artifactId>resilience4j-spring-boot2</artifactId>
<version>1.7.0</version>
</dependency>
For Gradle:
implementation 'io.github.resilience4j:resilience4j-spring-boot2:1.7.0'
Implementation
Now, let’s create a service that makes use of a circuit breaker to call a remote service:
import io.github.resilience4j.circuitbreaker.annotation.CircuitBreaker;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
@Service
public class LearnSpringBootOnlineService {
private final RestTemplate restTemplate;
public LearnSpringBootOnlineService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
@CircuitBreaker(name = "learnSpringBootOnlineService", fallbackMethod = "fallback")
public String callLearnSpringBootOnlineService() {
return restTemplate.getForObject("https://learnspringbootonline.com/api/service", String.class);
}
public String fallback(Throwable t) {
return "Fallback response: " + t.getMessage();
}
}
In this example:
- We have a LearnSpringBootOnlineService class that makes use of Spring’s
RestTemplate
to call a remote service. - The callLearnSpringBootOnlineService method is annotated with
@CircuitBreaker
. This annotation tells Resilience4j to create a circuit breaker named “learnSpringBootOnlineService” around this method. - The
fallbackMethod
attribute specifies the fallback method to be called when the circuit is open. - We define a fallback method named
fallback
that will be called when the circuit is open. This method returns a fallback response, which can be a predefined message or default value.
Now, you can use LearnSpringBootOnlineService
in your application to call remote services safely with circuit breaker protection:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication
public class LearnSpringBootOnlineApplication {
public static void main(String[] args) {
SpringApplication.run(LearnSpringBootOnlineApplication.class, args);
}
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
This setup allows you to make remote service calls using LearnSpringBootOnlineService.callLearnSpringBootOnlineService()
, and if the remote service fails, the circuit breaker will open, and the fallback
method will be invoked, providing a graceful degradation of service.
Configuration
When setting up a circuit breaker in a Spring Boot application, there are several configuration options available to customize its behavior according to the specific requirements of your application. Here are some of the key configuration options:
- Failure Thresholds: Configure the threshold for failures that trigger the circuit breaker to open. This includes the number of consecutive failures or the failure rate within a certain time window.
- Retry Strategy: Define how the circuit breaker handles retries after it has opened. You can specify a fixed wait time between retries or use an exponential backoff strategy.
- Timeouts: Set timeouts for remote service calls to prevent long-running requests from blocking the application. This includes both the connection and read timeouts.
- Fallback Mechanism: Define fallback methods or responses to be used when the circuit breaker is open or when requests fail. This ensures graceful degradation of service when the remote service is unavailable.
- Circuit Breaker State Management: Configure how the circuit breaker transitions between different states (e.g., closed, open, half-open) and the conditions for transitioning between these states.
- Metrics and Monitoring: Enable monitoring and reporting of circuit breaker metrics such as success rate, failure rate, and state transitions. This allows you to monitor the health and performance of your circuit breakers in real-time.
- Bulkhead Configuration: Configure the maximum number of concurrent requests allowed to a remote service to prevent overloading and resource exhaustion.
- Custom Event Handlers: Define custom event handlers to perform additional actions when specific events occur, such as when the circuit breaker opens or closes.
- Thread Pool Configuration: Customize the thread pool used by the circuit breaker for executing remote service calls and handling fallback methods.
These configuration options can typically be set either programmatically or through external configuration files (e.g., application.properties
or application.yml
) depending on the specific circuit breaker library being used (e.g., Resilience4j or Hystrix). By adjusting these configuration parameters, developers can fine-tune the behavior of circuit breakers to meet the resilience and performance requirements of their applications.
Conclusion
In conclusion, implementing a circuit breaker in a Spring Boot application using Resilience4j provides a robust solution for handling failures in distributed systems. By integrating the Resilience4j library and annotating methods with @CircuitBreaker
, developers can easily enhance the resilience and stability of their applications. The circuit breaker pattern helps to prevent cascading failures, gracefully handle errors, and improve overall system reliability. With proper configuration and fallback mechanisms, Spring Boot applications can efficiently manage calls to remote services, ensuring smoother operation even in the face of intermittent failures.
Explore our diverse collection of blogs covering a wide range of topics here.