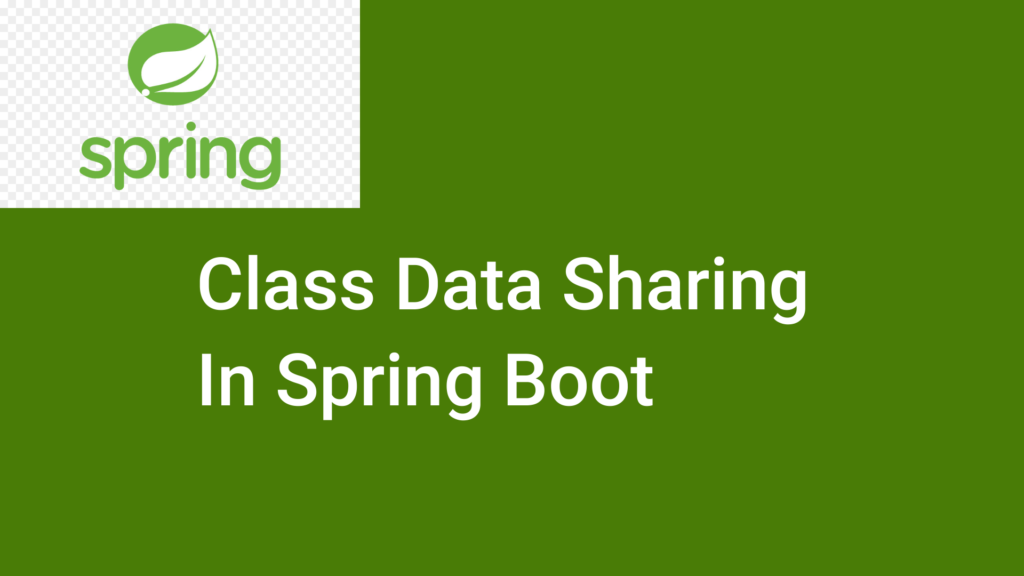
Table of Contents
Introduction To Class Data Sharing (CDS)
Class Data Sharing (CDS) is a feature in the Java Virtual Machine (JVM) that enhances the performance of Java applications by reducing startup time and memory usage. It does this by allowing the JVM to share class metadata (like class structure and constants) between multiple JVM instances. This shared metadata is stored in a shared archive file that can be mapped into memory when the application starts, reducing the need to re-load and re-process this data.
CDS Optimization in Spring Boot
In the context of Spring Boot applications, CDS optimization involves configuring your application to take full advantage of this JVM feature. By doing so, you can significantly reduce the time it takes for your Spring Boot application to start, which is particularly beneficial in environments that require rapid scaling or deployment, such as cloud-based microservices or serverless architectures.
Spring Boot 3.3 introduced better support for CDS by making it easier to create a CDS-friendly layout from the fat JAR file commonly used in Spring Boot applications. This involves breaking down the JAR into layers, which allows the JVM to efficiently use CDS to load only the necessary classes, further speeding up startup time.
Key Benefits
- Faster Startup Time: By using pre-loaded class metadata, the JVM can skip the class loading process, resulting in quicker application startups.
- Reduced Memory Usage: Sharing class metadata between JVM instances means less memory is needed for each instance, which is especially useful in resource-constrained environments.
- Improved Performance: Applications can perform better overall, especially in environments with high concurrency, as less time is spent on class loading.
Implementing CDS optimization in a Spring Boot application requires configuring your build process to generate the appropriate CDS archive and ensuring your deployment environment supports the necessary JVM features.
Implementation
To implement Class Data Sharing (CDS) optimization in a Spring Boot application, you need to follow a few steps. The process involves creating a CDS archive during the build process and then using that archive at runtime to speed up your application’s startup time.
Here’s how you can do it, You can generate a CDS archive by customizing the Spring Boot build process. Assuming you are using Maven, you can use the Maven Shade Plugin to create a JAR layout compatible with CDS.
Add the following configuration to your pom.xml
:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.3.0</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
<configuration>
<transformers>
<transformer
implementation="org.apache.maven.plugins.shade.resource.AppendingTransformer">
<resource>META-INF/spring.factories</resource>
</transformer>
</transformers>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
After building the project with the above configuration, you can create a CDS archive using the following command:
java -Xshare:dump -XX:+UseAppCDS -XX:SharedClassListFile=classlist.txt -XX:SharedArchiveFile=app-cds.jsa -cp target/your-app.jar
Once the CDS archive is created, you can run your Spring Boot application using the CDS archive to benefit from faster startup times:
java -Xshare:on -XX:+UseAppCDS -XX:SharedArchiveFile=app-cds.jsa -jar target/your-app.jar
-Xshare:dump
: This command generates the CDS archive.
-XX:+UseAppCDS
: Enables the use of the Application Class Data Sharing feature.
-XX:SharedClassListFile=classlist.txt
: Specifies the file that contains the list of classes to be included in the CDS archive.
-XX:SharedArchiveFile=app-cds.jsa
: Specifies the output file for the CDS archive.
-Xshare:on
: Ensures the JVM uses the CDS archive at runtime.
Conclusion
In conclusion, integrating Class Data Sharing (CDS) optimization into your Spring Boot application is a highly effective approach to enhancing performance, particularly in environments that demand rapid startup times and efficient resource utilization. By following the outlined steps—configuring your build process, generating a CDS archive, and running your application with this archive—you can significantly reduce startup latency and lower memory consumption.
This optimization is particularly valuable in modern deployment scenarios such as microservices and cloud-native applications, where every millisecond counts, and resource efficiency directly impacts scalability and cost. As the Spring ecosystem continues to evolve, leveraging JVM features like CDS in conjunction with Spring Boot 3.3 ensures that your applications are not only performant but also aligned with the latest best practices in Java development.
By mastering CDS optimization, you position yourself at the cutting edge of Spring Boot performance tuning, providing tangible benefits to your applications and a valuable edge in the competitive landscape of software development.
For more information refer https://docs.spring.io/spring-framework/reference/integration/cds.html or https://docs.oracle.com/javase/8/docs/technotes/guides/vm/class-data-sharing.html .
Explore our diverse collection of blogs covering a wide range of topics here.