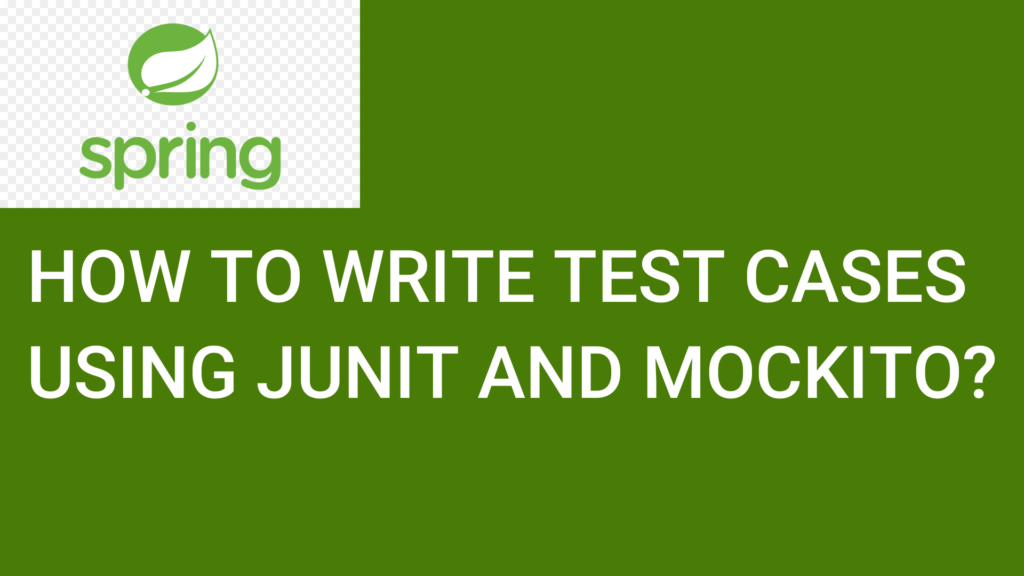
Table of Contents
Junit And Mockito Overview
JUnit is a popular testing framework for Java programming language. It provides annotations to identify test methods, assertions for verifying expected results, and other utilities for testing Java code. JUnit helps in automating the process of testing code, ensuring its correctness and reliability.
Mockito, on the other hand, is a mocking framework that allows you to create mock objects in your tests. Mock objects simulate the behavior of real objects in controlled ways, enabling you to isolate the code under test and verify interactions with dependencies.
Add Dependency
To use Mockito and JUnit in a Maven project, you need to include the following dependencies in your project.
Mockito
For Maven:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.11.2</version> <!-- Replace with the latest version -->
<scope>test</scope>
</dependency>
For Gradle:
testImplementation 'org.mockito:mockito-core:3.11.2' // Replace with the latest version
Junit
For Maven:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.8.2</version> <!-- Replace with the latest version -->
<scope>test</scope>
</dependency>
For Gradle:
testImplementation 'org.junit.jupiter:junit-jupiter-engine:5.8.2' // Replace with the latest version
Implementation
Here’s an example of writing test cases using JUnit 5 and Mockito in a Spring Boot application:
Let’s assume we have a simple service class LearnSpringBootOnlineUserService
that interacts with a repository to perform user-related operations:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class LearnSpringBootOnlineUserService {
@Autowired
private LearnSpringBootOnlineUserRepository learnSpringBootOnlineUserRepository;
public User getUserById(Long userId) {
return learnSpringBootOnlineUserRepository.findById(userId).orElse(null);
}
public User saveUser(User user) {
return learnSpringBootOnlineUserRepository.save(user);
}
public void deleteUser(Long userId) {
learnSpringBootOnlineUserRepository.deleteById(userId);
}
}
And we have a LearnSpringBootOnlineUserRepository interface:
import org.springframework.data.jpa.repository.JpaRepository;
public interface LearnSpringBootOnlineUserRepository extends JpaRepository<User, Long> {
}
Now let’s write test cases for the LearnSpringBootOnlineUserService
class using JUnit 5 and Mockito:
import static org.junit.jupiter.api.Assertions.*;
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.junit.jupiter.MockitoExtension;
@ExtendWith(MockitoExtension.class)
public class LearnSpringBootOnlineUserServiceTest {
@Mock
private LearnSpringBootOnlineUserRepository learnSpringBootOnlineUserRepository;
@InjectMocks
private LearnSpringBootOnlineUserService learnSpringBootOnlineUserService;
@Test
public void testGetUserById() {
// Mocking behavior of userRepository
User mockUser = new User(1L, "John Doe", "john@example.com");
when(learnSpringBootOnlineUserRepository.findById(1L)).thenReturn(java.util.Optional.of(mockUser));
// Calling the method under test
User result = learnSpringBootOnlineUserService.getUserById(1L);
// Verifying the result
assertNotNull(result);
assertEquals("John Doe", result.getName());
assertEquals("john@example.com", result.getEmail());
}
@Test
public void testSaveUser() {
// Mocking behavior of LearnSpringBootOnlineUserRepository
User userToSave = new User(null, "Alice", "alice@example.com");
User savedUser = new User(1L, "Alice", "alice@example.com");
when(learnSpringBootOnlineUserRepository.save(userToSave)).thenReturn(savedUser);
// Calling the method under test
User result = learnSpringBootOnlineUserService.saveUser(userToSave);
// Verifying the result
assertNotNull(result);
assertEquals(1L, result.getId());
assertEquals("Alice", result.getName());
assertEquals("alice@example.com", result.getEmail());
}
@Test
public void testDeleteUser() {
// Calling the method under test
learnSpringBootOnlineUserService.deleteUser(1L);
// Verifying that the deleteById method of LearnSpringBootOnlineUserRepository was called with the correct argument
verify(learnSpringBootOnlineUserRepository).deleteById(1L);
}
}
In these test cases, we use Mockito to mock the LearnSpringBootOnlineUserRepository
dependency of the LearnSpringBootOnlineUserServic
e. We then inject this mocked repository into the
using the LearnSpringBootOnlineUserServic
e@InjectMocks
annotation. Finally, we write test methods to test the functionality of the
methods, such as LearnSpringBootOnlineUserServic
egetUserById()
, saveUser()
, and deleteUser()
.
Conclusion
In conclusion, the test cases presented utilize JUnit 5 and Mockito to verify the functionality of a LearnSpringBootOnlineUserServic
e in a Spring Boot application.
- Mockito is employed to mock the
, allowing for controlled behavior during testing.LearnSpringBootOnlineUserRepository
- JUnit 5 provides the framework for defining test methods and assertions, ensuring the correctness of the tested functionality.
- Each test method follows a typical Arrange-Act-Assert pattern:
- Arranging the necessary preconditions and mock behavior,
- Acting by invoking the methods under test, and
- Asserting the expected results.
- Additionally, Mockito’s
verify()
method is utilized to confirm interactions with the mock repository.
These test cases offer a comprehensive approach to testing the
, ensuring its correctness and reliability in handling user-related operations within a Spring Boot environment.LearnSpringBootOnlineUserServic
e