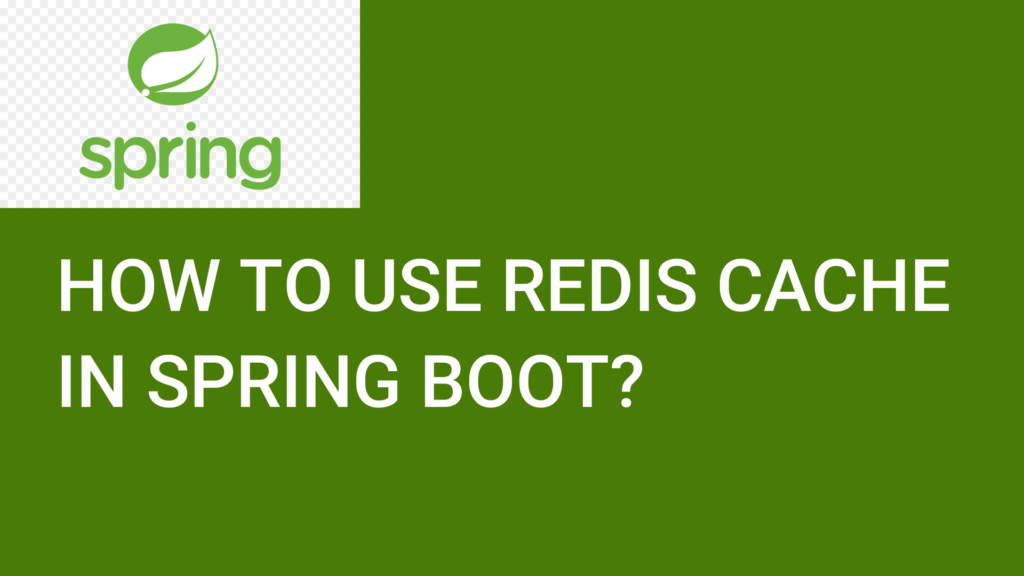
Table of Contents
Introduction
Redis is an open-source, in-memory data store that excels for caching purposes. It’s known for its speed, flexibility, and wide range of data structures it supports (strings, lists, sets, hashes, etc.). By caching frequently accessed data in Redis, your Spring Boot application can significantly improve performance:
- Reduced Database Load: By retrieving data from Redis instead of the primary database for repeated requests, you lessen the strain on your database.
- Faster Response Times: Data retrieval from RAM (where Redis resides) is much faster than querying a database on disk storage.
Add Dependencies
For Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
For Gradle:
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-cache'
implementation 'org.springframework.boot:spring-boot-starter-data-redis'
}
Implementation Of Redis Cache
- Configuration (Optional):
- Spring Boot auto-configures Redis with default settings if a Redis server is available.
- To customize, create a
RedisProperties
bean and configure properties like host, port, password, etc.
- @EnableCaching Annotation:
- Add
@EnableCaching
to a configuration class to enable caching functionality.
- Add
- @Cacheable Annotation:
- Annotate methods or classes that you want to cache results from. This instructs Spring Boot to:
- Check the Redis cache for a cached entry with the specified key (usually constructed from method arguments).
- If the entry exists and hasn’t expired, return the cached value.
- If no cached entry exists or it’s expired, execute the method, store the result in Redis, and return it.
- Annotate methods or classes that you want to cache results from. This instructs Spring Boot to:
@SpringBootApplication
@EnableCaching
public class LearnSpringBootOnlineApp {
@Autowired
private LearnSpringBootOnlineService learnSpringBootOnlineService;
@Cacheable("myCache") // Cache the result of this method
public String getData(String key) {
return learnSpringBootOnlineService.expensiveOperation(key); // Simulates a slow database call
}
public static void main(String[] args) {
SpringApplication.run(LearnSpringBootOnlineApp.class, args);
}
}
// LearnSpringBootOnlineService.java
public class LearnSpringBootOnlineService {
public String expensiveOperation(String key) {
// Simulate a slow database call (replace with your actual logic)
System.out.println("Performing expensive operation for key: " + key);
return "Data for key: " + key;
}
}
Connecting to the Cache Server
Spring Boot will automatically discover and connect to a running Redis server based on the following order:
- Environment Variables: Look for
REDIS_HOST
andREDIS_PORT
environment variables. - Application Properties: Check for
spring.redis.host
andspring.redis.port
properties. - Standalone Redis Server: If no configuration is provided, Spring Boot looks for a Redis server running on the default port (6379) on localhost.
For a custom Redis server setup, provide the necessary configuration details using environment variables or application properties.
In your Spring Boot application’s application.properties
file, you can set the spring.redis.host
and spring.redis.port
properties to configure the connection to your Redis cache server. Here’s how:
Setting the Properties
spring.redis.host=my-redis-server.example.com
spring.redis.port=6380 # Assuming your Redis server is listening on a non-default port
- Spring Boot attempts to auto-configure the Redis connection if a server is running on the default port (6379) on localhost. You only need to set these properties if your Redis server has a different configuration.
- If you need to specify additional configuration options, such as a password or database selection, Spring Boot provides other related properties under the
spring.redis
prefix. Refer to the Spring Boot documentation for details on these additional properties.
Conclusion
In conclusion, integrating Redis cache into your Spring Boot application offers a compelling approach to optimize performance. By caching frequently accessed data in Redis, you can significantly reduce database load and deliver faster response times to your users. The process involves adding essential dependencies, enabling caching with @EnableCaching
, and strategically utilizing annotations like @Cacheable
to manage caching behavior. Spring Boot streamlines the connection to your Redis server, making the setup efficient. Remember to consider expiration times and error handling for a robust caching strategy. By implementing Redis cache effectively, you can create a more responsive and scalable Spring Boot application.
Explore our diverse collection of blogs covering a wide range of topics here.